Goal:
This large assignment aims to give you experience with computational design. You will use computer programming in Processing to write algorithms and procedures with turtle geometry and L-systems to generate creative designs. Some of the work you create as part of this assignment will be fabricated (laser-cut) in the next assignment.
GitHub repository:
https://github.com/EldyLazaro/comp-fab/tree/main/week-4/
TASK 1: Processing functions
Use Processing functions to create a composite image with the simple geometries listed below in any colors (e.g., background, stroke, fill) of your choosing:
- Draw a point that is not at the origin.
- Use the above-generated point as a starting point to create a line.
- Draw a circle with a well-defined radius.
- Draw a triangle.
- Draw a rectangle (with unequal side lengths).
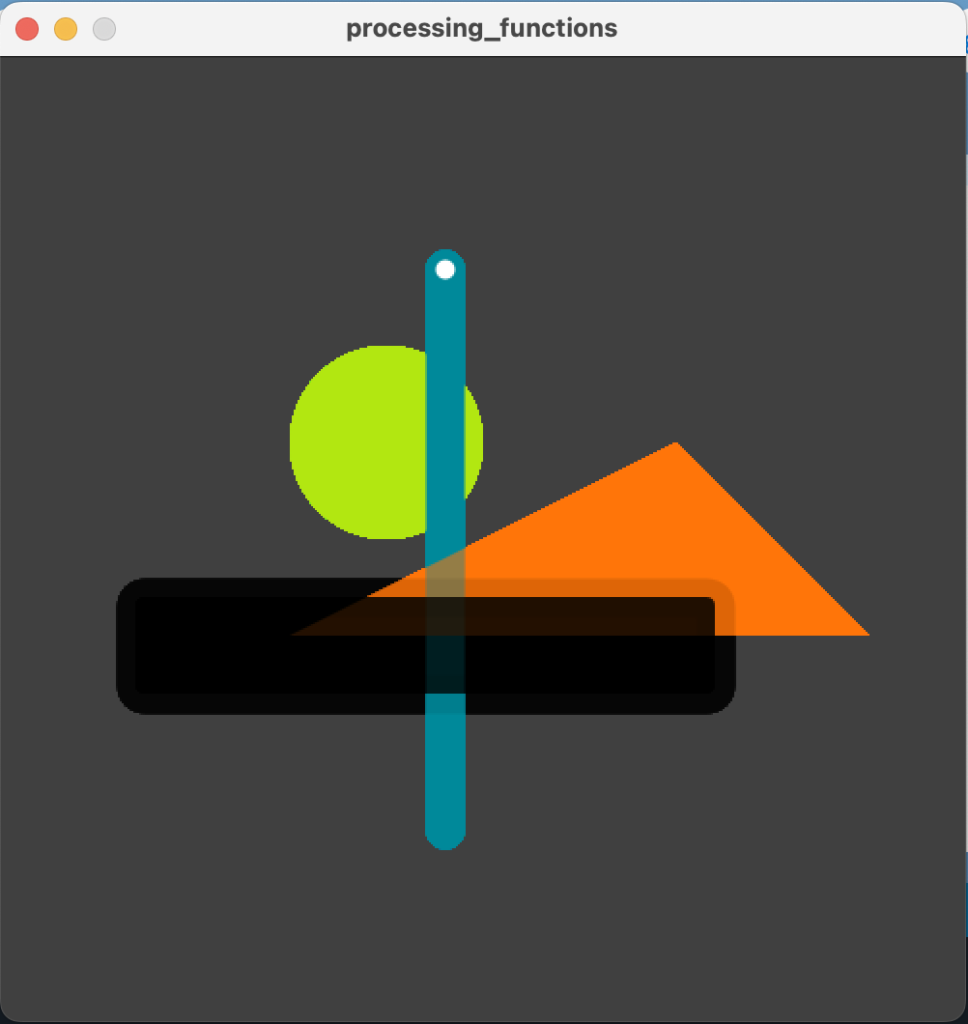
void setup() {
//setup code - gets called once
size (500, 500);
background (64);
}
void draw() {
// draw a green circle on the left
fill(#b2e711);
noStroke();
circle(200, 200, 100);
// draw a line
stroke(#00899a);
strokeWeight(20);
line(230, 110, 230, 400);
//draw a triangle behind the other shapes
fill(#ff7509, 148);
noStroke();
triangle(150, 300, 350, 200, 450, 300);
// draw a rectangle below without fill, just stroke
fill(0, 200);
stroke(5, 20);
rect(70, 280, 300, 50, 5);
//draw a point
stroke(255);
strokeWeight(10);
point (230, 110);
}
TASK 2: Turtle Library
Use the Turtle library (in Processing) to create the following geometries listed below:
- Draw the shapes for capitalized letters “ I “ and “T”. Ensure that you don’t overwrite over paths in order to create these shapes. Hint: You should use the turtle’s penUp and penDown functions.
- Draw a triangle using turtle movement.
- Draw a regular pentagon using the movement of the turtle. Recall that for a regular pentagon, each of the internal angles measures 108°. In general for a regular polynomial with a number of sides ‘k’, the internal angle is given by [(k-2)/k]*180°.
- Draw a circle with a well-defined radius using only turtle movement (do not use built-in functions in Processing).
See code on Github
TASK 3: L-system
- Define each of them by — the vocabulary (variables and constants), axiom, and production rules.
For each L-system, list the first three strings produced after the axiom (beyond iteration n=0).
- Use Processing, the Turtle Library, and LA1_LSystemBase (base code on GitHub) to program a representation of your system.
- In your implementation, be sure to use multiple variables and constants (“F“, “B“, “-“, “+“, and others, etc.) along with push/pop (e.g., “[“, “]“) to give your L-System a memory (see lecture slides for more details).
See code on Github